►Function Parameters
C51 make use of registers and memory locations for passing parameters. By default C function pass up to three parameters in registers and further parameters are passed in fixed memory locations. You can disable parameter passing in register using NOREGPARMS keyword. Parameters are passed in fixed memory location if parameter passing in register is disabled or if there are too many parameters to fit in registers.
►Parameter passing in registers
C functions may pass parameter in registers and fixed memory locations. Following table gives an idea how registers are user for parameter passing.
C51 make use of registers and memory locations for passing parameters. By default C function pass up to three parameters in registers and further parameters are passed in fixed memory locations. You can disable parameter passing in register using NOREGPARMS keyword. Parameters are passed in fixed memory location if parameter passing in register is disabled or if there are too many parameters to fit in registers.
►Parameter passing in registers
C functions may pass parameter in registers and fixed memory locations. Following table gives an idea how registers are user for parameter passing.
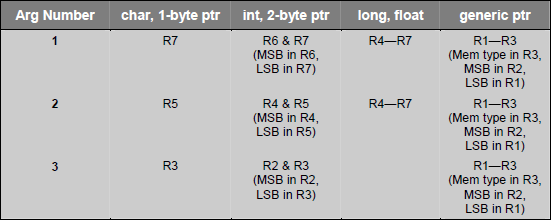
Following example explains a little more clearly the parameter passing technique:

Parameters passed to assembly routines in fixed memory lcoation use segments named
?function_name?BYTE : All except bit parameters are defined in this segment.
?function_name?BIT : Bit parameters are defined in this segment.
All parameters are assigned in this space even if they are passed using registers. Parameters are stored in the order in which they are declared in each respective segment.
The fixed memory locations used for parameters passing may be in internal data memory or external data memory depending upon the memory model used. The SMALL memory model is the most efficient and uses internal data memory for parameter segment. The COMPACT and LARGE models use external data memory for the parameter passing segments.
Function return values are always passed using CPU registers. The following table lists the possible return types and the registers used for each.
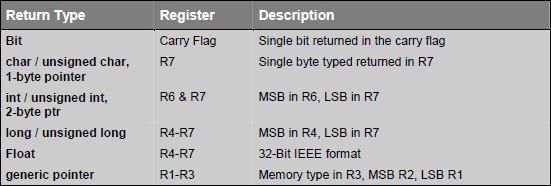
►Example
Following example shows how these segment and function decleration is done in assembler.
CODE:
;Assembly program example which is compatible
;and called from any C program
;lets say asm_test.asm is file name
name asm_test
;We are going to write a function
;add which can be used in c programs as
; unsigned long add(unsigned long, unsigned long);
; as we are passing arguments to function
;so function name is prefixed with '_' (underscore)
;code segment for function "add"
?PR?_add?asm_test segment code
;data segment for function "add"
?DT?_add?asm_test segment data
;let other function use this data space for passing variables
public ?_add?BYTE
;make function public or accessible to everyone
public _add
;define the data segment for function add
rseg ?DT?_add?asm_test
?_add?BYTE:
parm1: DS 4 ;First Parameter
parm2: ds 4 ;Second Parameter
;either you can use parm1 for reading passed value as shown below
;or directly use registers used to pass the value.
rseg ?PR?_add?asm_test
_add:
;reading first argument
mov parm1+3,r7
mov parm1+2,r6
mov parm1+1,r5
mov parm1,r4
;param2 is stored in fixed location given by param2
;now adding two variables
mov a,parm2+3
add a,parm1+3
;after addition of LSB, move it to r7(LSB return register for Long)
mov r7,a
mov a,parm2+2
addc a,parm1+2
;store second LSB
mov r6,a
mov a,parm2+1
addc a,parm1+1
;store second MSB
mov r5,a
mov a,parm2
addc a,parm1
;store MSB of result and return
;keil will automatically store it to
;varable reading the resturn value
mov r4,a
ret end
;and called from any C program
;lets say asm_test.asm is file name
name asm_test
;We are going to write a function
;add which can be used in c programs as
; unsigned long add(unsigned long, unsigned long);
; as we are passing arguments to function
;so function name is prefixed with '_' (underscore)
;code segment for function "add"
?PR?_add?asm_test segment code
;data segment for function "add"
?DT?_add?asm_test segment data
;let other function use this data space for passing variables
public ?_add?BYTE
;make function public or accessible to everyone
public _add
;define the data segment for function add
rseg ?DT?_add?asm_test
?_add?BYTE:
parm1: DS 4 ;First Parameter
parm2: ds 4 ;Second Parameter
;either you can use parm1 for reading passed value as shown below
;or directly use registers used to pass the value.
rseg ?PR?_add?asm_test
_add:
;reading first argument
mov parm1+3,r7
mov parm1+2,r6
mov parm1+1,r5
mov parm1,r4
;param2 is stored in fixed location given by param2
;now adding two variables
mov a,parm2+3
add a,parm1+3
;after addition of LSB, move it to r7(LSB return register for Long)
mov r7,a
mov a,parm2+2
addc a,parm1+2
;store second LSB
mov r6,a
mov a,parm2+1
addc a,parm1+1
;store second MSB
mov r5,a
mov a,parm2
addc a,parm1
;store MSB of result and return
;keil will automatically store it to
;varable reading the resturn value
mov r4,a
ret end
Now calling this above function from a C program is very simple. We make function call as normal function as shown below:
CODE:
extern unsigned long add(unsigned long, unsigned long);
void main(){
unsigned long a;
a = add(10,30);
//a will have 40 after execution
while(1);
}
void main(){
unsigned long a;
a = add(10,30);
//a will have 40 after execution
while(1);
}
You just copied and pasted the example. What is the bloody use?
ReplyDeleteWhat was needed was to explain the purpose of each item in the segment declaration and so forth. Perhaps you yourself do not understand and have never tried this code. Show me real world practical example, then I will believe that you know what you are talking about.
o996y5lhlrr974 vibrating dildos,sex toys,dildos,love dolls,wolf dildo,women sex toys,double dildos,horse dildo,Panty Vibrators w863w2wdbal854
ReplyDeleteq653d4ofjlk000 dildo,Bullets And Eggs,fantasy toys,Male Masturbators,dildos,penis rings,silicone sex doll,sex toys,sex chair j257g8ducxm057
ReplyDeletexk887 wholesale nfl jerseys,cheap jerseys,Cheap Jerseys from china,Cheap Jerseys free shipping,nfl jerseys,Cheap Jerseys free shipping,cheap nfl jerseys,wholesale nfl jerseys,nfl jerseys uh280
ReplyDelete